java graphics program案例 Create a graphics program to draw moving stars and other type of shapes.
java graphics program案例 科目Create a graphics program to draw moving stars and other type of shapes.
Ask user to input “how many objects do you want to create?“
draw (how many / 2) stars
draw (how many / 2) other shapes
apply collision detection to all the stars and shapes (right-left-top-bottom), and rebound after collision
You must use random class to set initial values of each shape (x, y, size, speedx, speedy, color, etc)
Sample code here:
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import java.util.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Star {
int x;
int y;
int size;
int speedX;
int speedY;
Color color;
}
public class cs210Star extends JFrame {
public static ArrayListmyStar = new ArrayList<>();
static int width = 800;
static int height = 600;
static int howMany = 30;
public cs210Star() { // Constructor (Ch08)
super("Your Title");
setBounds(100, 100, width, height); // at your monitor
setResizable(false);
setVisible(true);
setDefaultCloseOperation(EXIT_ON_CLOSE);
}
public static void createStar() {
Random rand = new Random();
int R, G, B;
for (int i = 0; i < howMany; i++) {
Star s = new Star();
R = rand.nextInt(256);
G = rand.nextInt(256);
B = rand.nextInt(256);
s.color = new Color(R, G, B);
s.x = rand.nextInt(width);
s.y = rand.nextInt(height);
s.size = rand.nextInt(10) + 10;
s.speedX = rand.nextInt(5) + 1;
s.speedY = rand.nextInt(5) + 1; myStar.add(s);
}
}
public void paint(Graphics g) {
g.setColor(Color.BLACK);
g.fillRect(0, 0, width, height);
for (int i = 0; i < myStar.size(); i++) { // AllayList Size
g.setColor(myStar.get(i).color); myStar.get(i).x += myStar.get(i).speedX; myStar.get(i).y += myStar.get(i).speedY;
drawStar(g, myStar.get(i).x, myStar.get(i).y, myStar.get(i).size);
}
try {
Thread.sleep(10);
} catch (Exception exc) {
}
repaint();
}
public void drawStar(Graphics g, int sx, int sy, int size) {
int[] xCoords = new int[10];
int[] yCoords = new int[10];
int ang = 90 - 36;
double rad;
double PI = 3.14159;
for(int i = 0; i < 10; i++) { if (i % 2 == 0) rad = size * 0.38; else rad = size; xCoords[i] += (int)(rad * Math.cos(PI * ang / 180.0));
yCoords[i] -= (int)(rad * Math.sin(PI * ang / 180.0));
ang += 36;
xCoords[i] += getX();
yCoords[i] += getY();
}
g.fillPolygon(xCoords, yCoords, 10);
}
public static void main(String[] args) { createStar();
new cs210Star();
}
}
java graphics program案例 cs代码案例
2020-02-18
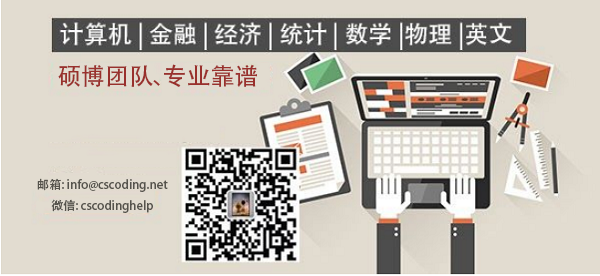