1 Introduction
You will be required to add features to the “Battleship” game created in Task 1 using the C programming language (in the C89 standard).
Your completed program will:
• Meet the gameplay requirements outlined in part one
• Read the ship locations from a text file (instead of using ship_locations.c)
• Use a linked list to store and replay a history each shot the player makes
• Allow the player to save a game (by saving the command history)
• Allow the player to load a game (by replaying the command history)
• Determine when a ship has been sunk
• Determine when the player has won the game by sinking all ships
This project is separated into multiple sections. Make sure to read each section carefully before starting.
2 Code Design
You must thoroughly document your code using C comments (/* ... */). For each function you define and each datatype you declare (e.g. using struct or typedef), place a comment immediately above it explaining its purpose, how it works, and how it relates to other functions and types. Collectively, these comments should explain your design.
Your code should be structured in a way that each file has a clear scope and goal. For example, “main.c” should only contain a main function, “game.c” should contain functions that handle and control the game features, and so on. Functions should be located in reasonably appropriate files and you will link them using the makefile. DO NOT put everything together into one single source code file. Make sure you use the header files and header guards correctly. Never include .c files directly.
Make sure you free all the memory allocated by the malloc() function. Use valgrind to detect any memory leak and fix them. Memory leaks might not break your program, but penalty will still be applied if there is any. If you do not use malloc, you will lose marks.
Keep in mind that it is possible to lose significant marks with a fully working program that is written messily, has poor structure, contains memory leaks, or violates many of the coding standards.
3 Task Details
3.1 File Input
Instead of using the “get_ship_location” function from task 1, you will need to replace that functionality with File IO code to read the data from a text file.
The text file will start with two integers that represent the size of the map, then there will be one ship provided on each subsequent line of the file. Some example text files are provided on Moodle.
The filename of the ship input file should be provided as a command-line argument using argc and argv. If an incorrect number arguments or a non-existent input file is provided, the program should exit with an error message.
This input file should be read at the start of the program to set up the initial state and then never opened again.
There is no limit to the number of ships that could be in the file, however, you can assume that they will fit in the bounds of the map and not overlap.
3.2 Tracking and Replaying Moves
Each time the player shoots a location on the map, that information should be saved in a dynamically allocated generic linked list.
A new option should be available in the user interface: “Replay” which will go through each of the moves the player has made up until that point, replaying them on the map.
3.3 Saving and Loading a Game
In addition to the “Replay” command, two other new user interface options should be available: “Save” and “Load”
The “Save” command should prompt the user to enter a filename and save the contents of the history linked list to a file.
The “Load” command should prompt the user to enter a filename and load the contents of the file into the history linked list and then replay the game in the file up to the current move.
3.4 Winning the Game
If all locations associated with a ship have been hit, that ship should be sunk and a message displayed on the screen.
If all ships have been sunk, a victory message should be displayed and the game should end.
3.5 Makefile
You should manually write a makefile according to the format explained in the lecture and practical. It should include Make variables, appropriate flags, appropriate targets, correct prerequisites, conditional compilation, and clean rule. We will compile your program through the makefile. If the makefile is missing, we will assume the program cannot be compiled, and you will lose marks.
4 Compilation
You must provide a single valid makefile for your project. When testing your code, the makefile will be used to compile it.
Warning: Failure to provide a makefile will result in zero (0) marks being awarded for compilation and it will be assumed that your code does not compile.
5 Report
If your program is incomplete or has errors, or if you have added new features, please submit a short report that outlines these issues or features. This report is not assessed but will aid in marking.
6 Submission
Once complete you are to submit your entire project electronically, via Moodle, before the submission deadline.
Your submission is to be a single .tar.gz file containing:
Your implementation – including all your source code (.c files, .h files, makefile, etc...).
Note that all pre-compiled code will be removed before marking.
Your report – A single PDF file containing everything mentioned in Section 5
You are responsible for ensuring that your submission is correct and not corrupted. You may make multiple submissions, but only your latest submission will be marked.
Standard late submission and extension policies will be applied as per college policy.
7 Demonstration
You will be required to demonstrate each of the worksheet submissions during your tutorial session. The final project submission will also be demonstrated in the tutorial session following the weeks of the submission date.
If you cannot attend your registered tutorial session you must make arrangements with the lecturer as soon as possible. You may be asked several questions about your project in this demonstration.
Failure to demonstrate your work will result in a mark of ZERO (0). During the demonstration you will be required to answer questions about your design. If you cannot answer the questions satisfactorily, you will also receive a mark of ZERO (0).
Battleship game
2023-01-03
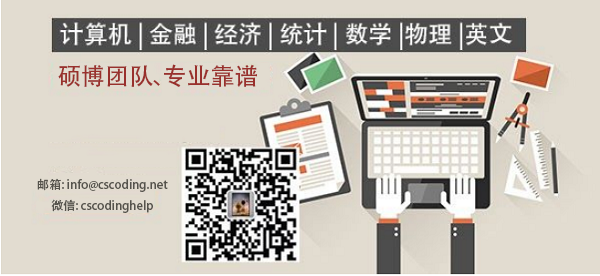