Overall Program
Omicrest Security Systems are manufacturers of smart cameras and home surveillance systems. Their smart surveillance systems are configured to use advanced machine learning techniques to detect objects such as packages and people that are recorded on security cameras. The system records video footage when an object is detected and logs information about the object as well as which of the eight cameras detected it. Your program is to read this information from a text file into one or more Linked List data structures and perform the required processing operations on the data. Your program should be compiled with make and contain a working makefile.
Task 1 – Data Types
Declare suitable C datatypes to represent each of the following sets of information in a header file.
1. A SecurityLog type that includes
• Date (The day, month and year when the data was collected)
• Log entries (A collection of one or more log entries)
2. A LogEntry type that includes:
• Start time (A UNIX Timestamp)
• End time (A UNIX Timestamp)
• Cameras (A character that acts as a set of 8 binary bit flags)
• Detected object (An Object – see below)
• Confidence value (A percentage)
3. An Object type that includes:
• ID (An integer)
• Name (Max 127 characters)
• Description (Max 255 characters)
Hint: Marks have been allocated for using a linked list Data structure
Task 2 – Loading Data
Write a function (or collection of functions) that will read a log file and populate the data types declared in Task 1.
SecurityLog data is saved in text based .txt files. There can be many security logs for different days in the same file with each SecurityLog delimited by an empty line.
A SecurityLog starts with the date for the log; Then the LogEntry and Object information appears on subsequent lines. The LogEntry data is delimited by $ symbols and the data is in the following format:
start_time$end_time
cameras
confidence
id$name$description
The following is a portion of the contents of a sample file available to download on Moodle:
2024-1-25
1706113669$1706113679
B
96.5
243$Package$Amazan Delivery
1706120865$1706120877
@
99.8
243$Package$Amazan Delivery
2024-1-26
1706238442$1706238493
B
93.3
646$Human$A person in a white shirt
Your function should:
• Take in a filename for the above data
• Dynamically allocate the structures you designed in Task 1.
• Read each of the SecurityLogs from the provided file.
• Read each of the LogEntries and detected Objects from the provided file.
• Return a pointer to a collection of LogEntry structures you designed in Task 1.
If the file cannot be opened, your function should return NULL. An error message is not required.
Task 3 – Processing the data
Write a function (or collection of functions) that will import a pointer to a SecurityLog. Thisfunction should go through each of the LogEntries and display the data contained within.
For each of the log entries, the program should display the date, object name, object description and the recording’s duration in seconds (calculated from the start and end times). It should only show entries that have objects that have been detected with a confidence value of over 60%.
Before moving on to the next log entry, the program should also display the information about which of the eight cameras detected the object using the “cameras” bit flags.
Each bit in the cameras value corresponds to one camera numbered from left to right.
For example:
If the cameras value is ‘B’ that is “10000010” in binary. This means that Camera 1 and Camera 7 are active.
Use binary operations to accomplish this.
The output printed to the user should be in a clear and human readable format.
Task 4 – Main
Write a main function that accepts a filename as a command-line parameter.
Your main function should:
• Use the loading code from Task 2 to read the contents of the provided file into a list.
• Select a SecurityLog at random from the list.
• Use the code from Task 3 to appropriately process the selected SecurityLog.
• If a file fails to read, print an error message and return 1, otherwise return 0.
• Perform all necessary freeing of dynamically allocated memory.
Task 5 – Shell Script
Write a bash script named SecurityCameras.sh that will perform the following operations in order.
1. Use make clean and make to rebuild and compile your program
2. Execute your program using the input file provided on Moodle
3. Search the output of the program for anyone wearing a “white shirt” or a “black
If your program does not return 0 when executed in step 2, the bash script should echo an error message.
Compilation and Makefile
Your code should compile using make and should have a well-structured and functioning makefile.
Coding Practices
Proper structuring of code with reasonable C files and header files (including header guards). Your code should be well structured, well commented and easy to read. As with any code for this unit, it is to abide by the Coding Standards that have been set from the start.
No Memory Leaks
Your code should free all dynamically allocated heap memory. 0 marks will be awarded if no heap memory is used.
C programming to read information from a text file
2023-01-04
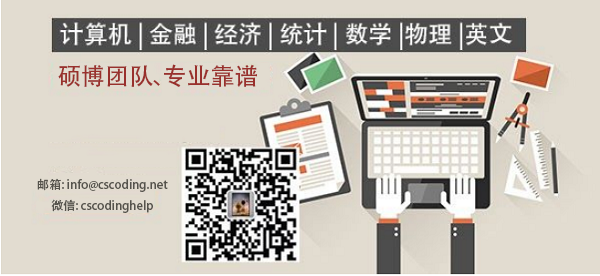