For Question 1, please download the file, and work with the existing code as instructed in the question.
To submit the , please create a Zip file with the respective answers, labelled by Question number, and send through in a private conversation between yourself and the instructor
案例
Question 1
The file named DistanceDifference.cpp contains an incomplete program that should be able to calculate the distance between a series of geocoded locations. Two parts of the program need to be completed:
1. The portion of the main() function that calculates the distances between each of the hard-coded 5 locations, and stores it in a two-dimensional array declared as distances.
2. A portion of the calculateDistanceBetweenLocations(l1, l2) function; omitted code spaces are designed with a // TODO: comment.
The code is properly commented in order for you to understand exactly what is being done, however you should not touch parts of the program that are not earmarked with a
// TODO: comment.
Question 2
Design and implement a Class called
Course –separated into its respective header (Course.h) and implementation file (Course.cpp).
The class contains a dependency on a Section structure, which should have the following
interface:
struct Section {
string section_code;// Section Code
string days[2];// Array of days section meets per week
string times[2];// Array of times section meets each day
int student_count;// Number of students in the section
}
The class should have the following private variables:
string course_name
long int course_id
string department
string instructor_name
Section *sections
int section_count
The class should have a default constructor that initializes the following variables to the specified values:
course_name = “”
course_id= -1
department = “”
instructor_name = “Baruch Faculty”
sections = nullptr
section_count = 0
The class should have the following public functions(The explanation of the function follows in italics):
· void setCourseInformation(string courseName, long int courseId, string department, string instructorName);
This function sets the class’ private variables to the values passed to the function
· void display CourseInformation() const;
This function outputs the course information, in the following format:
:by
· void addClassSection(string sectionCode, string days[], string times[], int studentCount);
This function adds a new section to an array referenced by the sections pointer variable,
and updates the section_count variable.
HINT: When adding a new section, remember that existing sections must be preserved.
· bool removeClassSection(string sectionCode);
This function removes an existing section from the sections array. It should ensure that
the section exists, and if it does it should return TRUE after being removed. If it does not
exist, the function should return FALSE
· int getSectionCount() const;
This function returns the number of sections attached to the course
code:
#include#include#include
using namespace std;
// Location Structure
struct Location {
// Name of Location
string name;
// Latitude of Location
double latitude;
// Longitude of Location
double longitude;
};
// Location Structure using Radians
struct LocationWRadians {
// Name of Location
string name;
// Latitude Radians
long double latitude;
// Longitude Radians
long double longitude;
LocationWRadians() {
name = "";
latitude = 0;
longitude = 0;
}
LocationWRadians(string n, long double lat, long double longi) {
name = n;
latitude = lat;
longitude = longi;
}
};
/**
* Calculates the distance between two geographic locations and returns this value
*
* @param Location l1 The first location
* @param Location l2 The second location
* @return double
*
*/
long double calculateDistanceBetweenLocations(Location l1, Location l2);
/**
* Displays Summary Table Header
*
* @param locations
* @param size
*/
void displaySummaryTableHeader(const Location locations[], int size);
/**
* Display Summary of Distances
* @param locations
* @param distances
*/
void displaySummaryTable(const Location locations[], const long double distances[5][5]);
/**
* Converts earthly degrees to radians
* @param degree
* @return long double
*/
long double toRadians(long double degree);
int main() {
// An array of five locations
const Location locations[5] = {
{"Moscow", 55.75, 37.62}, {"Calcutta", 22.6763858, 88.0495367},
{"Sao Paulo", -23.6815315,-46.8754883}, {"Buenos Aires", -34.6156625,-58.5033383},
{"New York", 40.6976701,-74.2598661}
};
// An two-dimensional array to hold the distance between each location
long double distances[5][5];
// Calculate distances between all locations and store them in the distances array declared above such that:
// each row of the array represents one of the locations AND
// each column of the array represents the set of distances between the row location and each other location
// HINT: You need two loops, one nested inside of the other. The calculateDistanceBetweenLocations function can be used
// to find the distance between two locations
// TODO: WRITE YOUR CODE BELOW
// END YOUR CODE
// Output Distances In A Table
displaySummaryTableHeader(locations, 5);
displaySummaryTable(locations, distances);
cout << endl << "Program ending..."; return 0; } /*********************************** ***** Function Implementations **** ***********************************/ void displaySummaryTableHeader(const Location locations[], int size) { cout << setw(15) << ""; for (int i = 0; i < size; i++) { cout << setw(20) << locations[i].name; } cout << endl; } void displaySummaryTable(const Location locations[], const long double distances[5][5]) { for(int i = 0; i < 5; i++) { cout << setw(15) << locations[i].name; for (int j = 0; j < 5; j++) { cout << setw(20) << ((distances[i][j] == 0.00) ? "" : to_string(static_cast(distances[i][j])));
}
cout << endl; } } long double toRadians(const long double degree) { long double single_degree = M_PI/180; return single_degree * degree; } // TODO: Complete Function long double calculateDistanceBetweenLocations(const Location l1, const Location l2) { // Convert the latitude and longitudes from degree to radians and store the radians version of the two locations // in the two new LocationWRadians objects, radians1 & radians2. // TODO: Modify code below. You may use the toRadians(degree) function to calculate the radians value, passing the function either a longitude or latitude value LocationWRadians radians1; LocationWRadians radians2; // Calculate Distance Using Haversine Formula // 1. Calculate difference between radians longitude of second location and first location long double longitude_difference = // TODO: Write Formula Here // 2. Calculate difference between radians latitude of second location and first location long double latitude_difference = // TODO: Write Formula Here // LEAVE CODE BELOW UNTOUCHED // Calculate a long double a = pow(sin(latitude_difference/2), 2) + cos(radians1.latitude) * cos(radians2.latitude) * pow(sin(longitude_difference/2), 2); // Calculate b long double b = 2 * asin(sqrt(a)); // Radius of Earth in // Kilometers, R = 6371 // Use R = 3956 for miles const long double R = 6371; // Multiple b * R and return result return b * R; }
案例CS:C案例设计和实现一个名为Course.h和course.c 加一个补全代码
2017-08-19
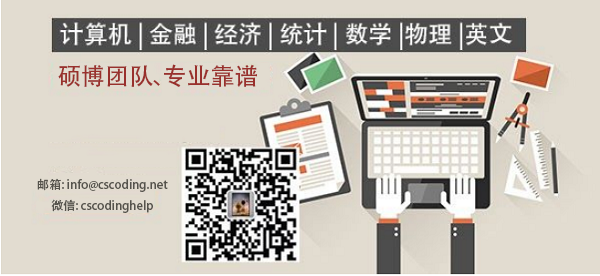