There are ten labs total in the course (C)CPS 209 Computer Science II taught by Ilkka Kokkarinen during the Spring 2018 term. The same ten labs are used in the daytime science transition program and in the Ryerson Chang School of Continuing Education.
Since many of these labs refer to the work done in the previous labs, you should create one BlueJ project folder named "209 Labs" into which you write all these ten labs. In this same project folder, you should also add the JUnit test classes provided by the instructor, and the text file warandpeace.txt of the classic novel known for its extreme length that is used as a "big data" (well, big enough for the era that it comes from) source for some of the JUnit tests for the labs. You should download and save this text file inside this project folder from the DropBox link. Do not edit this text file in any way, not even to just add or remove some little whitespace character.
Each of these ten labs is worth the same two points of your total course grade. For labs 5, 7 and 9 that ask you to write a Swing component, these two marks are given for these components passing the visual inspection of the behaviour specified in the lab. The other seven labs must cleanly pass the JUnit tester of that week to receive the two points for that lab. No partial marks whatsoever are given for labs that do not not pass these tests. In all computation, being 99% correct is same as being 0% correct. (And in fact, in many situations the former would be much worse.)
To compensate for this strict policy of correctness and to simplify the job for handling the labs in this double-paced course, in both daytime science transition course and evening Chang School course, all ten labs have the exact same deadline, the noon the day after the last lecture. Before that, there is no rush to complete any lab, so you can take your time to get each lab to work so that it will pass the JUnit tests. You can then simply come to each lab session to work on and get help for whichever lab you are currently working on.
In all these labs, silence is golden. Since many of these JUnit testers will test your code with a large number of pseudo-randomly generated test cases, your methods should be absolutely silent and print nothing on the console during their execution. Any lab that prints anything at all on the console during the execution will be unconditionally rejected and receive a zero mark.
You may not modify the JUnit testers provided by the instructor in any way whatsoever, but your classes must pass these tests exactly the way that your instructor wrote them. Modifying a JUnit tester to make it seem that your class passes that test, even though it really does not, is considered serious academic dishonesty and will be automatically penalized by the forfeiture of all lab marks in the course.
Once you have completed all the labs that you think you are going to complete before deadline, you will submit all your completed labs in one swoop as the entire "209 Labs" BlueJ project folder that contains all the source files and the provided JUnit testers. Even if you write your labs working on Eclipse or some other IDE, it is compulsory to submit these ten labs as one BlueJ project folder. No other form of submission is acceptable.
The students in Chang School should submit their completed project folders as email attachments to [email protected]. The students in the daytime transition program course will have the course TA set up a system for lab submissions, and they should not send their labs to the instructor.
ACCIDENTS HAPPEN, SO MAKE FREQUENT BACKUPS OF THE LAB WORK THAT YOU HAVE COMPLETED. SERIOUSLY. THIS CANNOT BE EMPHASIZED ENOUGH IN NOT JUST THIS COURSE, BUT IN ALL OUR LIVES IN THIS AGE OF COMPUTERS.
Lab 1
JUnit: PolynomialTestOne.java
In spirit of the Fraction ple class seen in the first lecture, your first task in this course is to implement the class Polynomial whose objects represent polynomials of variable x with integer coefficients, and their basic mathematical operations. (If your math skills on polynomials have become a bit rusty since you last used them back in high school, check out the page "Polynomials" in the "College Algebra" section of "Paul's Online Math Notes", the best and still very concise online resource for college level algebra and calculus that I know of.) As is good programming style unless there exist good reasons to do otherwise, this class will be intentionally designed to be immutable so that Polynomial objects cannot change their internal state after they have been constructed. The public interface of Polynomial should consist of the following instance methods.
@Override public String toString()
Implement this method first to return some kind meaningful, human readable String representation of this instance of Polynomial. This method is not subject to testing by the JUnit testers, so you can freely choose for yourself the textual representation that you want this method to produce. Having this method implemented properly will become immensely useful for debugging the remaining methods that you will write inside Polynomial class.
public Polynomial(int[] coefficients)
The constructor that receives as argument the array of coefficients that define the polynomial. For a polynomial of degree n, the array coefficients contains exactly n + 1 elements so that the coefficient of the term of order k is in the element coefficients[k]. For ple, the polynomial
5x3 – 7x + 42 that will be used as ple in all of the following methods would be given as the coefficient array {42, -7, 0, 5}.
Terms missing from inside the polynomial are represented by having a zero coefficient in that position. However, the coefficient of the highest term of every polynomial should always be nonzero, unless the polynomial itself is identically zero. If this constructor is given as argument a coefficient array whose highest terms are zeroes, it should simply ignore those zero terms. For ple, if given the coefficient array {-1, 2, 0, 0, 0}, the resulting polynomial would have degree of only one, as if that argument array had really been {-1, 2} without these leading zeros.
To guarantee that the Polynomial class is immutable so that no outside code can ever change the internal state of an object after its construction (at least not without resorting to underhanded tricks such as reflection), the constructor should not assign only the reference to the coefficients array to the private field of coefficients, but it must create a separate but identical defensive copy of the argument array, and store that defensive copy instead. This ensures that the stored coefficients of the polynomial do not change if somebody later changes the contents of the shared coefficients array that was given as the constructor argument.
public int getDegree()
Returns the degree of this polynomial. For ple, the previous polynomial has degree 3.
public int getCoefficient(int k)
Returns the coefficient for the term of order k. For ple, the term of order 3 of the previous polynomial equals 5, and the term of order 0 equals 42.
public int evaluate(int x)
Evaluates the polynomial using the value x for the unknown symbolic variable of the polynomial. For ple, when called with x = 2 for the previous ple polynomial, this method would return 68. Your method does not have to worry about potential integer overflows, but can assume that the final and intermediate results of this computation will always stay within the range of int.
Lab 2
JUnit: PolynomialTestTwo.java
The second lab continues with the Polynomial class from the first lab by adding new methods for polynomial arithmetic in its source code. (There is no inheritance or polymorphism yet in this lab.) Since the class Polynomial is designed to be immutable, none of the following methods should
modify the objects this or other in any way, but return the result of that arithmetic operation as a brand new Polynomial object created inside that method.
public Polynomial add(Polynomial other)
Creates and returns a new Polynomial object that represents the result of polynomial addition of the two polynomials this and other. This method should not modify this or other polynomial in any way. Make sure that just like with the constructor, the coefficient of the highest term of the result is nonzero, so that adding the two polynomials 5x10 – x2 + 3x and -5x10 + 7, each of them of degree 10, produces the result polynomial –x2 + 3x + 7 that has a degree of only 2 instead of 10.
public Polynomial multiply(Polynomial other)
Creates and returns a new Polynomial object that represents the result of polynomial multiplication of the two polynomials this and other. Polynomial multiplication works by multiplying all possible pairs of terms between the two polynomials and adding them together, combining the terms of equal rank together into the same term.
Lab 3
JUnit: AccessCountArrayListTest.java
In the third lab, you get to practice using class inheritance to create your own custom subclass versions of existing classes in Java with new functionality that did not exist in the original superclass. Your third task in this course is to use inheritance to create your own custom subclass AccessCountArrayListthat extends the good old ArrayListfrom the Java Collection Framework. This subclass should keep an integer count of how many times the methods get and set have been called. (One counter keeps the simultaneous count for both methods.)
You should override the get and set methods so that both of them first increment the access count and then call the superclass version of that same method (use the prefix super in the method call to force this), returning whatever result the superclass version returned. In addition to these overridden methods inherited from the superclass, your class should define the following two brand new methods:
public int getAccessCount()
Returns the current count of how many times the get and set methods have been called for this
object.
public void resetCount()
Resets the access count field of this object back to zero.
Lab 4
JUnit: PolynomialTestThree.java
In this fourth lab, we continue modifying the source code for the Polynomial class from the first two labs to allow equality and ordering comparisons to take place between objects of that type. Modify the class definition so that this class now implements Comparable. Then write the following methods to implement equality and ordering comparisons.
@Override public boolean equals(Object other)
Returns true if the other object is also a Polynomial of the exact same degree as this, so that the coefficients of this and other polynomial are pairwise equal. If the other object is anything else, this method returns false. (You can actually implement this method last after implementing the method compareTo, since at that point its logic will be a one-liner after the instanceof check.)
@Override public int hashCode()
Whenever you override the equals method in a subclass, you should also override the hashCode method to ensure that two objects that their equals method considers to be equal will also have equal hash codes. This method computes and returns the hash code of this polynomial, used to store and find this object inside some instance of HashSet, or some other hash table based data structure. This hash code is computed by adding up the coefficients of this polynomial, but so that the coefficient for term k is multiplied by the element in position k % 5 in the array [547, 619, 877, 1013, 1163]. Define that array inside your class as a named constant MULTIPLIERS.
public int compareTo(Polynomial other)
Implements the ordering comparison between this and other polynomial, as required by the interface Comparable, allowing the instances of Polynomial to be sorted or stored inside some instance of TreeSet. This method returns +1 if this is greater than other, -1 if other is greater than this, and 0 if both polynomials are equal in the sense of the equals method.
A total ordering relation between polynomials is defined by the rule that any polynomial of higher degree is automatically greater than any polynomial of lower degree, regardless of their coefficients. For two polynomials whose degrees are equal, the result of the order comparison is determined by the highest-order term for which the coefficients of the polynomials differ, so that the polynomial with a larger such coefficient is considered to be greater in this ordering.
Be careful to ensure that this method ignores the leading zeros of high order terms if you have them inside your polynomial coefficient array, and that the ordering comparison criterion is precisely the one defined in the previous paragraph.
Lab 5
In this lab, your task is to create a custom Swing GUI component Head that extends JPanel. To practice working with Java graphics, this component displays a simple human head that, to also practice the Swing event handling mechanism, reacts to the mouse cursor entering and exiting the surface of that component. You should copy-paste a bunch of boilerplate code from the ple class ShapePanel into this class. Your class should contain one field a private boolean mouseInside, and the following methods:
public Head()
The constructor of the class should first set the preferred size of this component to be 500 by 500 pixels, and then give this component a decorative raised bevel border using the utility method BorderFactory.createBevelBorder. Next, this constructor adds a MouseListener to this component, this event listener object constructed from an inner class MyMouseListener that extends MouseAdapter. Override both methods mouseEntered and mouseExited inside your event listener class to first set the value of the field mouseInside accordingly and then call repaint.
@Override public void paintComponent(Graphics g)
Renders some kind of image of a simple human head on the surface of this component. This is not an art course so this head does not need to look fancy, but a couple of simple rectangles and ellipses suffice. (Of course, interested students of more artistic bent might want to try out more complicated shapes from the package java.awt.geom to produce a more aesthetically pleasing image.) If the value of the field mouseInside is true, the eyes should be drawn to be open, whereas if mouseInside is false, the eyes should be drawn to be closed. (As long as the eyes are somehow noticeably visually different based on the mouse entering and exiting the component surface, that is enough for this lab.)
To admire your reactive Swing component on the screen, write a separate HeadMain class that contains a main method that creates a JFrame that contains four separate Head components arranged in a neat 2-by-2 grid using the GridLayout layout manager.
Lab 6
JUnit: WordCountTest.java
This lab lets you try out reading in text data from the given BufferedReader and performing computations on that data. To practice working in a proper object-oriented framework, we design an entire class hierarchy that reads in text one line at the time and performs some operation for each line. The subclasses can then decide what that operation is by overriding the template met
案例:CS案例之java案例(C)CPS 209 Labs, Spring 2018 加急
2018-12-27
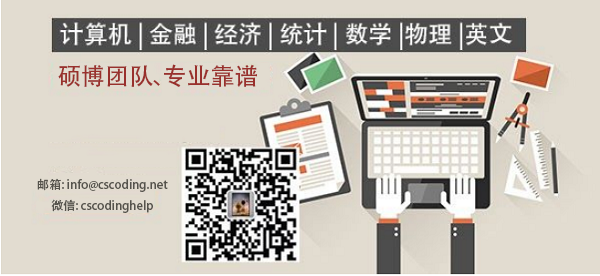