project # 2
Can be solved individually or in groups of 2 students
Create a zip file containing the required deliverables with all files containing the students names and numbers ( one submission per team)
Q1) Creating the stack classes
You are required to write your own generic stack implementation in Java that you will use in questions 2 and 3. (30 marks)
a. Create a stack interface Stackthat uses a generic type T and has the following abstract methods:
– isEmpty, isFull, peek (returns T), pop(returns T), void push(T), void clear() to clear the contents of the stack0, and int size (returns the number of elements in the stack).
– void reverse() reverses the contents of the stack ( in your implementation you can use an additional local stack in your method to help you with the reverse operation.)
– void display() prints out the contents of the stack (Hint: you can obtain the contents of your stack elements using toString())
b. Write your two stack implementations (ArrayStackand ListStack) using the stack interface.
c. Your stack implementations must have all instance variables as private.
d. Both implementations must a constructor that initializes the capacity of the stack. For the ListStack, the stack is full when the size reaches the initial capacity.
e. You must also implement public String toString() in both classes. It returns a String representation of the stack contents.
c. Create a simple main class QuestionOne that creates two stacks of Integers of capacity=20 one that uses the ArrayStackand the other uses the ListStackimplementations. Push 20 values in each: in the first (1,2,3,…,20) and in the second (20, 19,..,13,.., 1). Call the following methods for both stacks in the given order: display, reverse, display, peek, pop, pop, reverse, size, isFull, isEmpty, display, clear, display, isEmpty.
Deliverable: folder Q1
Stack.java
ArrayStack.java
ListStack.java
QuestionOne.java
Q2) Simple calculator (35 marks):
You are to design a simple calculator using the ArrayStack implementation in Q1 to perform additions, subtractions, multiplications and divisions. The user may enter an arithmetic expression in infix using numbers (0 to 9), parentheses((,),{,},[,],<,>) and the four arithmetic operations (+, -, *, /) only.
The first step to do so is to create a utility class MyCalculator that will have the following methods: a) Input of an expression and checking Balanced Parenthesis:
public static Boolean isBalanced(String expression)
This is a static method that will read a string representing an infix ((operand operation operand)) mathematical expression with parentheses from left to right and decide whether the brackets are balanced or not. For ple, (5*{7+22} is not valid, but (5*{7+22}) is valid.
To discover whether a string is balanced each character is read in turn. The character is categorized as an opening parenthesis, a closing parenthesis, or another type of character. Values of the third category are ignored for now. When a value of the first category is encountered, the corresponding close parenthesis is stored in the stack. For ple, when a “(“ is read, the character “)” is pushed on the stack. When a “{“ is encountered, the character pushed is “}”. The topmost element of the stack is therefore the closing value we expect to see in a well balanced expression.
When a closing character is encountered, it is compared to the topmost item in the stack. If they match, the top of the stack is popped and execution continues with the next character. If they do not match an error is reported (display a message about the error and return false). An error is also reported if a closing character is read and the stack is empty. If the stack is empty when the end of the expression is reached then the expression is well balanced.
For this application a parenthesis can be one of the following:
-parantheses: ( ) – angle brackets: < >
-curly braces: { } -square brackets: [ ] These must be defined as constants in the class.
b) Infix to Postfix conversion
Write a method that converts Infix expressions to postfix expressions using your stack implementation:
public static String infixToPostfix(String infix)
The method only takes balanced expressions with balanced parenthesis. The return is a string that has the Postfix expression of the input string.
c) Evaluating a Postfix expression
Write a method that evaluates a postfix expression: public static double evaluate(string postfix);
d) Your main class QuestionTwo
To evaluate your methods create the class QuestionTwo It must contain a public static void main(String[] args) method to run your code. This main method should ask the user to input one infix
expression per line until the user types “q” or “Q”. After every input, it should first test if the expression has balanced paranthesis and tells the user if the expression is balanced or not.
If the expression is balanced it will convert it into a postfix expression, displays the expression and then evaluates it and outputs the results and then ask for the next input.
If the expression is not balanced it tells the user that and asks for a new input.
Some rules:
-You should assume no whitespace in the infix expression provided by the user. For ple, your code should work for inputs like “2+(3×2)”. The postfix that you will print, however, should have tokens separated by whitespace. For ple, “3×21” should have the postfix
“3 21 ×”, and not “321×”. Here is a suggested part of your main that you can use
import java.util.Scanner; public class QuestionTwo{
public static void main(String[] args)
{
Scanner calcScan = new Scanner(System.in); Boolean finished=false;
while (!finished)
{
System.out.println("Enter a postfix expression or q to quit: "); String expression = calcScan.nextLine(); System.out.println(expression);
expression.trim();//omits leading and trailing whitepsaces System.out.println(expression);
if (expression.equalsIgnoreCase("q"))
{
finished=true;
} else
{//use your MyCalculator methods here
}
}
}
}
Deliverables:
– MyCalculator.java
-QuestionTwo.java
Q3) Towers of Hanoi (35 marks):
You are required to design an algorithm which solves the Tower of Hanoi puzzle (see the figure below). The objective of the puzzle is to move n discs from one tower to another, but with some rules in place as described
CS案例之JAVA Assignment 基础CS加急案例
2018-09-09
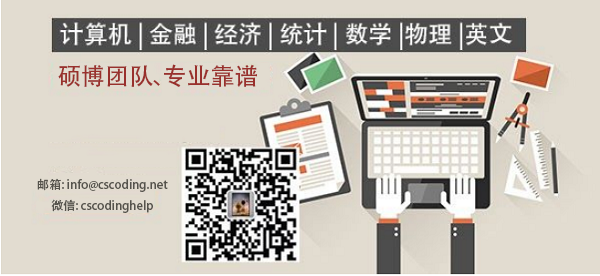