Notes
· Please follow both the "Programming Standards" and "project Guidelines" for all work you submit. Programming standards 1-22 are in effect, but see also the notes at the bottom of this project.
· Hand-in will be via the UM Learn project facility, which will be demonstrated in class. Make sure you leave enough time before the deadline to ensure your hand-in works properly.
· Official test data will be provided several days before the project due date. Until then, work with your own test data.
· All projects in this course will carry an equal weight.
project 1: An Online Ticket Exchange
Description
Getting tickets for games for certain sports teams can be difficult. Many tickets are already held by season ticket owners. Online fraudsters take advantage of this demand to sell phony tickets. However, owners of legitimate tickets may need to sell tickets to certain games and would like to do so without the inconvenience of having to arrange a meeting with a potential buyer.
Online ticket exchanges have been created by teams in order to protect both buyer and seller, and to make the process simple. Season ticket holders are the only ones who may offer tickets for sale, and they are automatically given an account on the exchange (they may also buy additional seats). Those wanting to buy tickets create an account on the exchange. When they wish to buy seats, they enter their buy request, and the exchange attempts to match them up with a seller. If a match is found, the exchange processes payment (we are not modelling the payment in this project) and makes the tickets available to the buyer, either as a printable ticket or a QR code that can be displayed on a smartphone.
Data Files
There are several data files containing different types of information. They are described below. Note that where names are specified, they may include spaces and special characters (e.g., single quotes) but do not include newline characters.
· Games file: this file contains information on the season's games, one game per line, including game number, date and name of the opponent, respectively. The game number is a positive integer, the date is written in yyyy/mm/dd format (where y, m and d are digits), and opponent is a name. An ple is shown below:
1 2018/09/4 Toronto Maple Leafs
· Season ticket file: this file contains information on the season ticket holders, one ticket holder per line. Included is the ticket holder's name, which may contain blanks, and the number of tickets held. An ple is shown below:
Chipman, Mark 12
· Transaction file: This file contains information on transactions that occur. Each transaction is on one line of the file. More details about transactions follow.
For each type of transaction, you may assume that the type of each data item is correct (i.e. integers or strings). Other types of errors are permitted though (e.g. incorrect spelling, or an integer not found in the data).
Each transaction begins with one of five 3-letter codes. A comment line is also supported.
1. ADD: Add a new ticket buyer to the exchange.
The line has the form ADD name where name is the name of the buyer. You may assume that buyer names are unique.
2. SEL: A season ticket holder offers tickets for sale.
The line has the form SEL game quantity name where game is the game number, quantity is how many tickets are for sale and name is the name of the ticket holder. When tickets are offered for sale, they must be assigned AT RANDOM to any buyers waiting for them.
3. BUY: A buyer offers to buy tickets.
The line has the form BUY game quantity name where game is the game number, quantity is how many tickets are wanted and name is the name of the buyer. A buyer may have only one BUY request for any single game.
4. CAN: A buyer cancels an offer to buy tickets.
The line has the form CAN game name where game is the game number and name is the name of the buyer.
5. REM: A buyer removes her account from the exchange.
The line has the form REM name where name is the name of the buyer. Only buyers can be removed from the exchange, and any outstanding buy offers must also be deleted.
6. #: Lines beginning with a hashtag are comments and are ignored by the program.
Transaction error processing
Each transaction may be either successful or contain one or more errors that must be reported. The list below describes some error conditions your program should detect (but there may be others as well).
1. ADD: name is already in the exchange as a buyer or ticket holder.
2. SEL: game is not a valid game number, quantity is more than the number owned by the ticket holder, name is not a ticket holder.
3. BUY: game is not a valid game number, name is not a member of the exchange, or already has a BUY request for this game.
4. CAN: game is not a valid game number, name is not a member of the exchange or else does not have a BUY request for this game.
5. REM: name is not a buyer in the exchange (you can't remove a ticket holder).
Main method and File.java
In order to make marking projects easier, your main method MUST accept the names of the three input files transaction file as a command-line argument. The data file names on the command-line must be in the same order as described above (games, ticket holders, transactions). The other two input files can have their names hard-coded into your program (as games.txt and ticketHolders.txt). A sample main class will be given to you, that has the code necessary to accept file names on the command-line, which you are free to use (or ignore) as you wish. Utility functions used by this sample main class are contained in File.java, which is also given to you.
NO PACKAGES
DO NOT USE PACKAGES! Any project that uses packages will receive a zero!
Output
For each transaction, produce a line of output (to standard output), describing the relevant details.
At the end of the program, produce a summary description. This description contains a list of all the ticket purchases for all games, ordered by game number first, then ordered by buyer.
More details of the output will be released shortly.
Data Structures
There are some restrictions on data structures you must use for the project. Do not use ANY built-in Java collection classes (e.g. ArrayLists, Hashes, …) in this project, or Java Generics: any linked structures must be your own, built from scratch. You should avoid using arrays other than one exception listed specifically below (other than for minor things such as temporary storage when parsing strings, etc.). See the notes at the bottom of this project for further details on arrays.
The dataset descriptions above should be enough for you to generate your own test data to start with. Official test data will be provided several days before the project is due.
Notes
· This is a large programming problem with many pieces to the puzzle – remember your incremental development strategy from COMP1020 and get small pieces working rather than trying to tackle the whole thing at once.
· The ADTs required above must be linked structures written by you. Do not use built-in Java collection classes anywhere here. Plain arrays (not ArrayLists) may be used as basic temporary storage to support things like string parsing using
split(). Don't use them to build data structures or for storage in data. If your solution requires ADTs other than those described above, these must also be your own linked structures.
· See the documentation for class Random in Java for built-in routines to choose randomly among the possibilities you generate.
· Your program will be tested by the markers under Unix and must run with a simple javac/java as per the "Running Java" instructions on the course website.
· As yet, we do not know much OO – you should follow the rules of abstraction and object-oriented programming based on what you have learned in previous courses and given the limitations specified in this project. Use separate classes for entities like buyers and offers to sell. Try to make other entities and classes for your ADTs. Do not be afraid of using many classes – breaking things up usually makes for much simpler pieces. The model solution I will show you uses more than ten classes, just as an ple.
CS案例之Java Programming 2018 Mini Project 案例CS之JAVA
2019-05-01
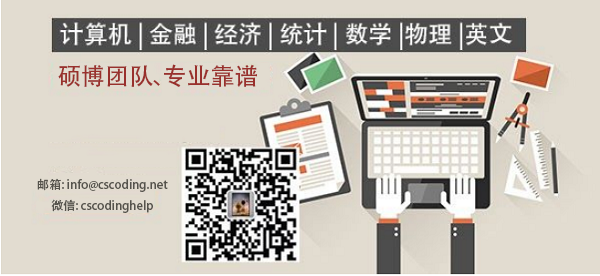