Asteroids Shooter
Description. You will implement an AI player to play a simplified version of Asteroids. In this version, the ship is anchored to the origin and can only rotate clockwise (CW) or counterclockwise (CCW). The asteroids are modeled as circles, as is the ship (the triangular ship is displayed for aesthetic purposes only). Your ship can turn xor fire each frame, where the goal is to accumulate as many points as possible, while also avoiding collisions with asteroids. For each game, your ship will be allocated one “shield”, which gives your ship immunity for a period of 200 frames. While the shield is active, you are unable to fire. You should not get killed with an unused shield.
Ship capabilities. Your ship class must provide the following three methods:
# Called to create a new player
def __init__(self, fieldSz, dt, turnRate, bulletSpeed, bulletRepeat, bulletRad, astSpeeds, astDiams,
totalFrames, points):
# Called when a new game of asteroids is started.
def newGame(self):
# Called to get the player's next command.
#
# The return value must be a 2-tuple
# of the form (fire, dir). Where dir = {negative, 0, positive} to indicate turn CCW, don't
# turn, or turn CW. fire is a boolean value to indicate whether to fire a
# bullet. If this is True, a bullet will be fire, but only if the bullet repeat
# rate is not exceeded. To execute the "shield", return None
#
# The inputs are:
# asteroids -- array of MovingObjects, one for each asteroid on screen
# heading -- the ship's heading (0 degrees is down +x), degrees.
# framesToFire -- num non-firing frames needed before next bullet.
def getCommand(self, asteroids, heading, framesToFire):
Updating rules. The getCommand method is called at the start of each frame. When it returns, the following sequence of events is performed:
· Gather information about current position/velocity of asteroids
· cmd = player.getCommand (asteroids, shipHeading, framesToFire)
· Perform the ship’s AI-determined action
· Update the position of asteroids and bullets
· Check if bullets intersect asteroids, remove asteroids that are hit. Bullets can hit only one asteroid.
· Update scoreboard
· Check if game over (asteroids intersect with ship or maximum number of frames reached)
Game parameters. The following parameters are defined by the system. Default values are shown in bold. You may tune your system to these particular values; however, the effectiveness of your software is increased if it can adapt to changes to one or more of these parameters:
· FIELD_SZ (700) – The dimensions of the playing area (will be square – 350×350). The origin is in the center of the playing area.
· NUM_FRAMES (2000) – Number of frames in entire game.
· DT (0.05) – Time for each frame
· AI_TIME (0.05) – Maximum amount of time your AI can take to issue command.
· INIT_NUM_ASTEROIDS (10) – Number of asteroids spawned to start the game
· TURN_RATE (4) – Number of degrees the ship turns in one frame.
· BULLET_REPEAT(8) – How many empty frames required between bullets
· BULLET_SPEED(200) – Speed of bullets.
· BULLET_RAD (2) – Radius of bullets
· INIT_AST_SPEED (100) – Speed of the large asteroid.
· AST_SPEED_FACTOR (1.5) – Multiplier for speed of next smaller asteroid: 100, 150, then 225
· INIT_AST_RAD (80) – Radius of the large asteroids.
· AST_SZ_FACTOR (0.5) – Multiplier for radius of next smaller: 80, 40, then 20
· SHIP_RAD (8) – integer radius of ship
· PTS ([2, 4, 10, -1]) – 4 integers indicating the score for the large, medium, and small asteroids. Last integer indicates cost of each shot.
Timing constraints. For the purposes of game play (updating ship, asteroids, bullets etc), will be assumed to take no time to execute. getCommand should not take longer than 0.05s (i.e., AI_TIME) on a PC typical of those in 006 Benton.
Baseline experiments. The starter code will execute your player over 20 specific games. This is accomplished using a list of 20 seeds for the random number generator. Thus, every team will be subjected to the same 20 configurations of asteroids. In your report, list your scores for these twenty games.
Simplifications. You are allowed to make simplifications to the game if you find the need. For example, you may assume: the ship is point-size, shooting cost zero, etc. Of course, such simplifications will not receive full credit, but may allow you to make progress if you find too many obstacles attempting the full requirements. In your report, identify all simplifications that you have made.
Notes.
· Do not modify any code main.py.
· Do not use any random number in your code.
· Bullets are removed once they move off the screen.
· The function dumpAsteroids (in ShipAI.py) can be used to dump all attributes of the asteroids to the screen.
· Examples of AI shooters were demonstrated the first day of class (one is clearly more effective than the other):
Scoring and additional requirements.
(85) Quality of design and implementation.
· (65) Basic operation
o Can accurately shoot asteroids.
o Can turn to face threats and then shoot
o Use shield to avoid a collision with an asteroid. Your AI should use the shield only when needed. Further, you should not have a collision with an unused shield.
o The AI operates within time constraints, with at most a few exceptions.
· (20) Advanced operation
o Intelligently prioritize threats to maximize score
o Effectively shoot at non-threats to maximize score
o Your algorithm should be robust under different game parameters. That is, your AI should behave reasonably under bullet speed, bullet life, etc.
(15) Writeup. You should include a 1 page write-up of what you have done. This should include a description of the techniques used in your intelligent player. In addition, describe techniques tested but not included in the final implementation. You may assume the reader has a level of knowledge equal to that of classmate’s. Your write-up should include a self-assessment of your player’s strengths and weaknesses. On a second page, include a table showing your scores for the 20 baseline games defined in main.py.
CS案例之Python Game游戏机器人对战:Homework Asteroids Sho
2018-02-17
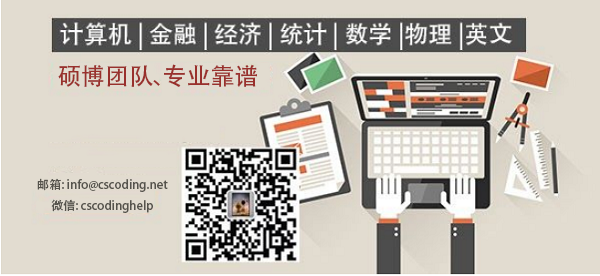